一.基本使用
1.Java多线程有两种方式,一种为继承Thread
类,一种为实现Runnable
方法
public class Main {
public static void main(String[] args) {
//方式一,继承Thread
Thread1 thread1 = new Thread1();
thread1.start();
//方式二,实现Runnable接口
Thread2 thread2 = new Thread2();
Thread thread = new Thread(thread2);
thread.start();
//使用匿名内部类实现Runnable接口
new Thread(new Runnable() {
@Override
public void run() {
System.out.println(Thread.currentThread().getId());
}
}).start();
//使用lambda表达式,即对匿名内部类的简化
new Thread(()->{
System.out.println(Thread.currentThread().getId());
}).start();
}
}
class Thread1 extends Thread{
public void run(){
System.out.println(Thread.currentThread().getId());
}
}
class Thread2 implements Runnable{
public void run(){
System.out.println(Thread.currentThread().getId());
}
}
2.join()
函数的理解:当线程A运行时调用另一个线程B的join方法时,线程A需等待线程B运行完毕才能继续join()方法后的语句,而效果就相当于当前运行线程”join”了一个线程进来,由于程序的顺序执行,当前线程只有运行完join的语句后才能继续运行后续语句
3.yield()
函数的理解:当前线程调用yield()方法则说明此线程放弃CPU控制权,自身转为就绪态,而转为就绪态意味着其可能再次被CPU选中从而转为运行态
二.java线程池
public ThreadPoolExecutor(int corePoolSize, int maximumPoolSize, long keepAliveTime, TimeUnit unit, BlockingQueue<Runnable> workQueue) {
this(corePoolSize, maximumPoolSize, keepAliveTime, unit, workQueue,
Executors.defaultThreadFactory(), defaultHandler);
}
参数含义:
corePoolSize:核心线程数
maximumPoolSize:最大线程数
keepAliveTime:线程存活保持时间
unit:线程活动时间的单位
workQueue:阻塞队列
解释:
当有线程进入线程池时若当前线程数少于corePoolSize则直接创建线程运行,若大于corePoolSize则进入workQueue等待corePoolSize运行完毕,若workQueue已满且线程数少于maximumPoolSize则创建临时线程,当临时线程空闲时间超过 keepAliveTime 则此线程销毁,若超过maximumPoolSize则抛出异常,图解过程如下图所示。
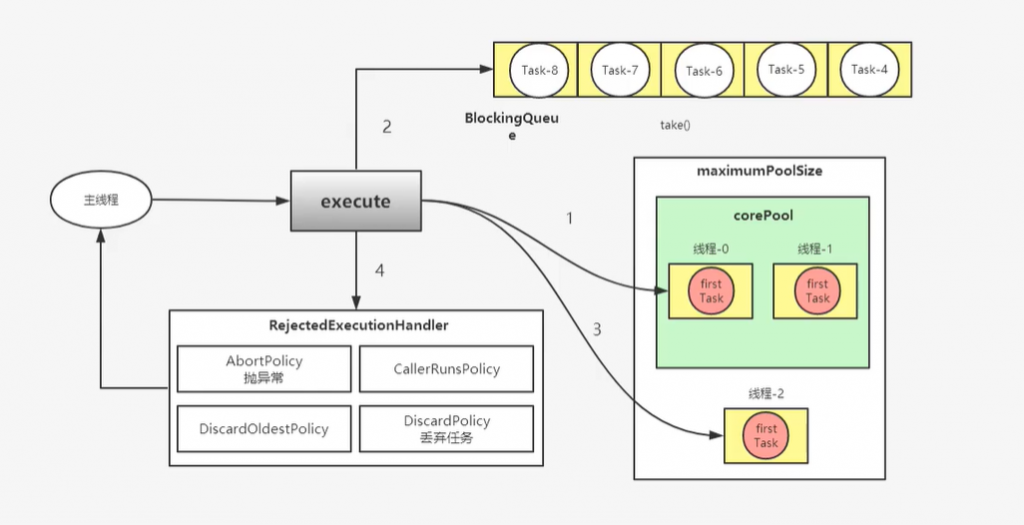
实例(对应上图图解):
public class Main {
public static void main(String[] args) {
ThreadPoolExecutor threadPoolExecutor = new ThreadPoolExecutor(
corePoolSize:2,
maximumPoolSize:3,
keepAliveTime:60,
TimeUnit.SECONDS,
new ArrayBlockingQueue<Runnable>(capacity:5)
);
for(int m=0;m<8;m++){
threadPoolExecutor.execute(new Thread1());
}//若大于8则抛出异常
threadPoolExecutor.shutdown();
}
}
class Thread1 implements Runnable{
@Override
public void run() {
System.out.println(Thread.currentThread().getName());
}
}
Comment